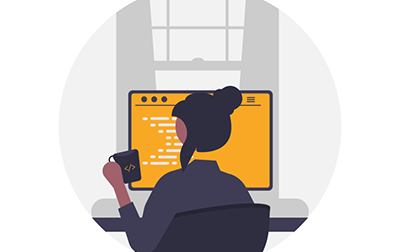
Python Training for Scientists and Engineers (PYT401)
Our Python Training for Scientists and Engineers course covers a wide range of topics, from fundamental concepts to advanced programming techniques and data manipulation. It's designed for beginners and experienced developers looking to enhance their Python skills and expand their knowledge in various Python libraries and tools.
The course begins with The Python Environment, introducing you to starting Python, using the interpreter, running scripts, and understanding Python on different operating systems. You'll also learn about various Python editors and IDEs to enhance your coding efficiency.
In Getting Started, you'll explore variables, built-in functions, strings, numbers, and type conversions. This module also covers essential concepts like writing output, string formatting, and command-line parameters.
Flow Control delves into managing program logic with conditional statements, relational and boolean operators, and loops. You'll learn how Python uses whitespace for code structure and explore different ways to exit loops.
The module on Lists and Tuples introduces Python's sequence types, including lists and tuples. You'll learn about indexing, slicing, iteration, and functions applicable to all sequences, as well as advanced topics like list comprehensions and generator expressions.
In Working with Files, you'll learn how to handle file input/output, including reading from and writing to text files, and working with binary data.
Dictionaries and Sets explores these essential data structures. You'll learn when and how to use dictionaries, iterate through them, and work with sets for efficient data storage and manipulation.
The Functions module teaches you to define and use functions, manage parameters, understand variable scope, return values, and work with lambda functions.
Exception Handling covers Python's error management capabilities, including syntax errors, exceptions, and how to handle them with try
, except
, else
, and finally
clauses.
You'll explore OS Services through the os
module, managing environment variables, executing external commands, and navigating file systems, including walking directory trees and handling dates and times.
The module on Modules and Packages introduces Python's modular structure, including initialization code, namespaces, documentation, and the use of imports to manage complex projects effectively.
In Classes, you'll learn object-oriented programming with Python, including defining classes, constructors, instance methods, attributes, inheritance, and multiple inheritance.
Programmer Tools explores tools like pylint
for code quality, unit testing with the unittest
module, debugging, benchmarking, and profiling Python applications to optimize performance.
The course also covers working with Excel Spreadsheets using the openpyxl
module to read, create, and modify spreadsheets.
XML and JSON teaches you how to create and parse XML files, use XPath for navigating XML, and read and write JSON data, essential for working with APIs and data exchange formats.
The iPython and Jupyter module introduces interactive computing tools, covering the basics of iPython and Jupyter for enhanced data analysis and visualization.
NumPy explores Python's scientific stack, focusing on creating and manipulating arrays, understanding array shapes, slicing, indexing, and leveraging NumPy's powerful mathematical functions.
You'll dive into SciPy, learning about its packages and functions for scientific computing, including practical examples for numerical analysis.
The module on pandas provides a deep dive into this powerful data manipulation library, covering Series, DataFrames, reading and writing data, and merging and joining datasets.
Finally, in matplotlib, you'll learn to create plots, customize styles, visualize data in various formats, and save images, providing powerful tools for presenting your data effectively.
- Create and run basic Python programs.
- Design and code modules and classes.
- Implement and run unit tests.
- Use benchmarks and profiling to speed up programs.
- Work with various data formats.
- Process XML and JSON.
- Manipulate arrays with NumPy.
- Get a grasp of the diversity of subpackages that make up SciPy.
- Leverage pandas to easily create and structure data.
- Use matplotlib to create amazing visualizations.
- Use Jupyter notebooks for ad hoc calculations, plots, and what-if?.
- The Python Environment
- Starting Python
- Using the Interpreter
- Running a Python Script
- Python Scripts on Unix
- Python Scripts on Windows
- Python Editors and IDEs
- Getting Started
- Using Variables
- Built-in Functions
- Strings
- Numbers
- Converting among Types
- Writing to the Screen
- String Formatting
- Command Line Parameters
- Flow Control
- About Flow Control
- What's with the White Space?
- if and else
- Conditional Expressions
- Relational Operators
- Boolean Operators
- while Loops
- Alternate Ways to Exit a Loop
- Lists and Tuples
- About Sequences
- Lists
- Tuples
- Indexing and Slicing
- Iterating through a Sequence
- Functions for All Sequences
- Operators and Keywords for Sequences
- Nested Sequences
- List Comprehensions
- Generator Expressions
- Working with Files
- Text file I/O
- Opening a Text File
- Reading a Text File
- Writing to a Text File
- "Binary" (Raw, or Non-delimited) Data
- Dictionaries and Sets
- About Dictionaries
- When to Use Dictionaries
- Creating Dictionaries
- Iterating through a Dictionary
- About Sets
- Creating Sets
- Working with Sets
- Functions
- Defining a Function
- Function Parameters
- Variable Scope
- Returning Values
- Lambda Functions
- Exception Handling
- Syntax Errors
- Exceptions
- Handling Exceptions with Try
- Handling Multiple Exceptions
- Handling Generic Exceptions
- Ignoring Exceptions
- Using else
- Cleaning Up with finally
- Re-raising Exceptions
- Raising a New Exception
- OS Services
- The os Module
- Environment Variables
- Launching External Commands
- Paths, Directories, and Filenames
- Walking Directory Trees
- Dates and Times
- Modules and Packages
- Initialization code
- Namespaces
- Executing modules as scripts
- Documentation
- Packages and name resolution
- Naming conventions
- Using imports
- Classes
- Defining Classes
- Constructors
- Instance methods and data
- Attributes
- Inheritance
- Multiple Inheritance
- Programmer Tools
- Program Development
- Comments
- pylint
- Customizing pylint
- Unit Testing
- The unittest Module
- Creating a Test Class
- Establishing Success or Failure
- Startup and Cleanup
- Running the Tests
- Debugging
- Benchmarking
- Profiling Applications
- Excel Spreadsheets
- openpyxl module
- Reading an Existing Spreadsheet
- Creating a Spreadsheet
- Modifying a Spreadsheet
- XML and JSON
- Creating XML Files
- Parsing XML
- Tags and XPath
- Reading and Wiritng JSON
- iPython and Jupyter
- About iPython and Jupyter
- iPython Basics
- Jupyter Basics
- NumPy
- Python's scientific Stack
- NumPy Overview
- Creating Arrays
- Creating Ranges
- Working with Arrays
- Shapes
- Slicing and Indexing
- Indexing with booleans
- Stacking
- Iterating
- Tricks with Arrays
- Matrices
- Data Types
- NumPy Functions
- SciPy
- About SciPy
- SciPy Packages
- SciPy Examples
- pandas
- About pandas
- Series
- DataFrames
- Reading and Writing Data
- Indexing and Slicing
- Merging and Joining Data Sets
- matplotlib
- Creating a plot
- Commonly Used Plots
- Customizing Styles
- Ad hoc data visualization
- Advanced Usage
- Saving Images
Each student will receive a comprehensive set of materials, including course notes and all the class examples.
Experience in the following would be useful for this Python class:
- While there are no programming prerequisites, programming experience is helpful. Students should be comfortable working with files and folders, and should not be afraid of the command line.
Live Private Class
- Private Class for your Team
- Live training
- Online or On-location
- Customizable
- Expert Instructors