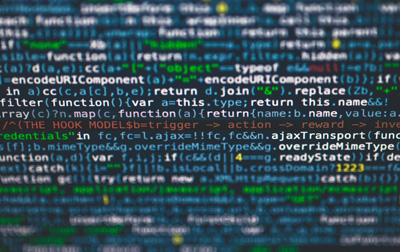
Objective-C for Experienced Programmers (OBJC102)
Objective-C is the language for developing iPhone and iPad applications. For people with prior object-oriented programming experience, this accelerated class teaches the syntax of the Objective-C language and the basics of the foundation classes. This includes user-defined objects, an in-depth review of the foundation classes for numbers, strings, arrays, dictionaries and dates, and an overview of the Objective-C memory management model. At the end of this programming language overview, students will understand the Objective-C language concepts required for building iPhone and iPad applications.
- Learn the underlying programming language for building iPhone and iPad applications.
- Learn the basics of the Objective-C language.
- Understand the memory management model for Objective-C and options for the developer.
- Learn about the Foundation classes for data manipulation and their use in Objective-C programming.
- Objective-C Overview
- The Xcode IDE
- Projects
- Hello World Application
- Objective-C
- Language Features
- Brief History
- Role in Mobile Device Applications
- The Xcode IDE
- Variables
- Numeric Variables
- Numeric Representations
- Integers
- Floating Point
- Comments
- Nonnumeric Variables
- char
- boolean
- Reference Variables
- The * and & Operators
- Variable Scope
- Numeric Variables
- Arithmetic
- Arithmetic Operators
- Addition and Subtraction
- Multiplication, Division, and Modulus
- Shorthand Notation
- Type Casting
- Math Library Functions
- pow
- random
- Arithmetic Operators
- Conditional Logic and Looping
- Conditional Statements
- Basic if Statement
- if else Statement
- switch Statement
- The Ternary Operator
- Looping Statements
- while Statement
- do...while Statement
- for Statement
- Conditional Statements
- Functions
- Purpose of a Function
- Declaration
- Header
- Body
- Calling a Function
- Passing Parameters by Value
- Passing Parameters by Reference
- Functions vs. Methods
- Object-oriented Programming According to Objective-C
- Object-oriented Programming (OOP)
- How Objective-C Implements OOP
- Encapsulation of Member Variables
- The Class Definition: Interface
- The Class Interface and @property
- Method vs. Function Syntax
- The Class Implementation and @synthesize
- Creating an Object from a Class
- Sending Messages to Objects
- The Role of Methods
- Visibility of Variables
- Inheritance
- Purpose of Inheritance
- Implementing Inheritance
- The Root Super Class
- Creating and Processing the Subclass
- NSObject Class
- Memory Acquisition
- alloc
- init
- Method Override
- Where's Abstraction?
- Polymorphism
- The Purpose of Polymorphism
- Polymorphism in Objective-C
- Placing Objects in Collections
- Using NSMutableArray
- Runtime Identification of Objects
- Arrays
- The "Classic" C Array
- Defining the Array
- Processing the Array
- Foundation Framework Arrays
- NSArray
- NSMutableArray
- The "Classic" C Array
- Foundation Framework
- The Foundation Framework
- Strings
- Date/Time
- Numbers
- Collections
- NSString
- Replacing char*
- Initialization
- NSString Methods
- NSNumber and NSNumberFormatter
- Replacing int, float, and more
- Initialization
- NSUInteger and NSInteger
- Formatting
- NSDate and NSDateFormatter
- NSDate
- NSDateFormatter
- The Foundation Framework
- Memory Considerations
- Memory Management in Objective-C
- Manual Retain-release
- Automatic Reference Counting
- Garbage Collection
- Strong and Weak
- Release and Retain
- Atomic and Nonatomic
- Automatic Reference Counting
- Memory Management in Objective-C
Each student will receive a comprehensive set of materials, including course notes and all the class examples.
Experience in the following is required for this Objective-C class:
- Object-oriented programming experience.
Experience in the following would be useful for this Objective-C class:
- c++ or C# experience.
Live Private Class
- Private Class for your Team
- Live training
- Online or On-location
- Customizable
- Expert Instructors