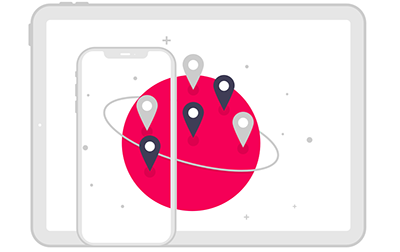
Programming RESTful Services with REST/JAX-RS (RES111)
This course provides a comprehensive introduction to building RESTful services using Java API for RESTful Web Services (JAX-RS). It is designed for developers who are looking to gain expertise in creating RESTful APIs, covering the fundamentals of REST architecture, JAX-RS framework, and advanced topics like security and versioning. The course offers practical, hands-on experience in building scalable and efficient RESTful services.
The course begins with an Introduction to REST and JAX-RS, where you'll learn the core principles of RESTful services, including statelessness, resource identification, and representational state transfer. You'll explore the benefits of REST over traditional web services, the HTTP methods commonly used in REST (GET, POST, PUT, DELETE), and how JAX-RS simplifies the development of RESTful services using annotations and conventions.
Next, you'll dive into Setting Up Your Development Environment, guiding you through installing and configuring the necessary tools such as Java, Maven, and an IDE like Eclipse or IntelliJ. You'll learn how to set up a JAX-RS project and create a basic RESTful service, establishing a solid foundation for the more advanced concepts covered later in the course.
The Resource Design module introduces the design principles of RESTful services, including defining resources, URIs, and how to map HTTP methods to CRUD operations. You'll learn best practices for structuring URIs, managing resource hierarchies, and working with path parameters, query parameters, and form data. This section emphasizes designing APIs that are easy to use, maintain, and scale.
In the Working with HTTP Status Codes and Headers section, you'll explore how to handle various HTTP responses, such as successful requests, client errors, and server errors. You'll learn to set and read custom HTTP headers, manage content negotiation, and implement proper error handling using JAX-RS exceptions. Practical exercises will reinforce your understanding of managing client-server communication effectively.
The Request and Response Handling module focuses on processing incoming requests and structuring responses in your RESTful service. You'll learn to use JAX-RS annotations to map request data to Java objects, manage different content types like JSON and XML, and utilize response builders to format your API outputs consistently.
Data Binding with JAXB and JSON covers how to serialize and deserialize data between Java objects and XML/JSON formats. You'll work with JAXB annotations for XML binding and explore libraries like Jackson for JSON processing. This module ensures that your RESTful services can communicate effectively with various data formats commonly used in web APIs.
Security in RESTful Services introduces authentication and authorization techniques to protect your APIs. You'll learn about basic and token-based authentication methods, securing endpoints using JAX-RS filters, and implementing role-based access control (RBAC). The module also covers HTTPS and securing data in transit, helping you build services that safeguard sensitive information.
The Asynchronous Processing module covers how to handle long-running tasks and improve the performance of your RESTful services using asynchronous methods. You'll learn to implement non-blocking operations in JAX-RS, enabling your APIs to respond faster and scale better under heavy loads.
Versioning and Documentation of RESTful Services teaches you how to version your APIs to manage changes over time. You'll learn strategies for URI versioning, content negotiation, and backward compatibility. This module also covers tools like Swagger for documenting your APIs, making it easier for developers to understand and integrate your services.
The course concludes with Advanced JAX-RS Features, where you'll explore features like filters, interceptors, and exception mapping. You'll also learn about hypermedia as the engine of application state (HATEOAS) and how to implement hypermedia-driven APIs. Practical exercises will help you apply these advanced concepts to build robust and flexible RESTful services.
By the end of this course, you'll have the skills to design, build, and maintain RESTful services using JAX-RS, enabling you to create scalable and secure APIs that integrate seamlessly with other applications. You'll be prepared to tackle real-world challenges in building RESTful web services and enhance your skills as a backend developer.
- Understand the purpose and role of web services in general, and how they are architected to expose business systems and processes over the web
- Understand the concepts and principles of REST and HTTP applications
- Expanded knowledge of HTTP, including its full set of methods and their intended uses, important headers, response codes, and content types
- Understand REST APIs, including resource identifiers and the URI namespace, resources and subresources, and WADL
- Understand configuration, deployment, and the runtime environment, including per-request and singleton objects, options for dependency injection, etc.
- Understand how HTTP requests get dispatched to service methods
- Understand content negotiation and the importance of Accept and Content-Type headers, and how they impact method dispatching
- Bind request inputs to method parameters, including path parameters, query parameters, and headers
- Exchange business data by communicating in HTTP entities in both XML and JSON format
- Handle errors using Java exceptions and appropriate HTTP response codes
- Learn how to integrate JAX-RS services with other Java EE technologies like servlets, EJB, and CDI, and how JAX-RS fits into the larger Java EE landscape
- Write browser clients using Ajax-JavaScript
- Write Java clients using the JAX-RS 2.0 Client API, including standalone clients and server-side components invoking remote services
- Learn how to secure RESTful resources
- Web Services Overview
- Definition
- Legacy Systems
- Benefits of Web Services
- Architecture
- Standards and Portability
- XML and Related Standards
- JSON
- HTTP
- SOAP-Based Services
- Overview
- SOAP Messages, Requests, and Responses
- WSDL
- Java APIs and Programming Models
- Definition
- Introduction to REST
- Overview and Principles
- REST Characteristics
- Resources and Operations
- REST Principles
- Requests and Responses
- REST APIs
- URI Templates
- GET, POST, PUT, DELETE
- Safe and Idempotent Methods
- Comparison of REST and SOAP
- Overview and Principles
- Introduction to JAX-RS
- APIs and Implementations
- JAX-RS Overview, Annotations
- JAX-RS Implementations
- Runtime Environment
- Application Server, Servlet-Only Container
- Architectural and Implementation Perspectives
- Configuring the Application
- Applications, Resources, and Providers
- JAX-RS Applications
- Resource Classes and @Path
- Provider Classes and @Provider
- Default Lifecycles
- The Application Class and rest-path
- Ajax-JavaScript Clients
- Overview
- Classic vs. Ajax Interactions
- Working with Ajax-JavaScript
- APIs and Implementations
- Resources and Requests
- Resources and Subresources
- Root Resource Classes, Resource Methods, Subresource Methods
- @GET, @POST, @PUT, @DELETE
- Subresource Locators
- Naming Conventions and Rules
- Dispatching Requests to Methods
- Binding Request Data
- Request Data Injection and Conversion
- Default Values
- Fields vs. Method Parameters
- Context-Based Injection
- Injection via @Context
- Context-Injectable Types
- Context Injection from the Web Container
- Fields vs. Method Parameters
- Resources and Subresources
- HTTP Entities
- Complex Content and Entities
- Working with Complex Content
- @Consumes and @Produces
- Content Negotiation
- Standard Entity Providers
- Working with JSON
- Returning Data as JSON
- Working with JSON in JavaScript
- Processing JSON Responses
- Working with XML
- JAXB and Mapping to XML
- Returning Data as XML
- Working with XML on the Client
- Customizing Content, Custom Media Types
- Working with Collections
- XML vs. JSON
- Complex Content and Entities
- Responses
- Response Class
- Return Types and HTTP Response Codes
- Appropriate Responses for HTTP Methods
- Choosing the Right Response
- Error Handling
- Exception Mappers
- WebApplicationException
- Response vs. Thrown Exception
- Error Responses
- Subresource Locators
- Motivation and Uses
- Locating the Locator
- Initializing the Subresource
- Binary Content
- File, InputStream, StreamingOutput
- Using StreamingOutput
- Response Class
- Java Client API
- Java Client - Options and Ingredients
- Building and Sending the Request
- Consuming the Response
- Options for the Response Data
- Asynchronous Requests
- Integration with Java EE
- Integration with EJB
- CDI - Contexts and Dependency Injection
- Activation, Scopes, and JAX-RS Lifecycles
- Injection in CDI-Enabled JAX-RS Applications
- Enhanced Java EE Lifecycle
- Security
- Java EE Security Overview
- Security Requirements in JAX-RS
- Declarative, Role-Based Security
- Security Constraints
- Annotation-Based Security
- Authentication
- Configuration
- Authentication Models: Basic, Digest, Client-Cert
- Programmatic Security
- SecurityContext
- Client Security
- HTTPS
- Java EE Security Overview
Each student will receive a comprehensive set of materials, including course notes and all the class examples.
Experience in the following is required for this Java class:
- Attendees must be experienced in Java and ideally will be somewhat familiar with XML, Java web applications, and other Java EE standards.
Experience in the following would be useful for this Java class:
- Familiarity with Ajax, JavaScript, and JSON.
Courses that can help you meet these prerequisites:
Live Private Class
- Private Class for your Team
- Live training
- Online or On-location
- Customizable
- Expert Instructors