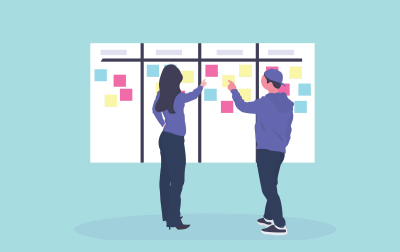
MySQL Development Training (MYS201)
This course provides a comprehensive guide to MySQL development, covering essential skills for working with relational databases, writing SQL queries, and developing stored programs. Designed for database developers, data analysts, and IT professionals, this course equips you with the knowledge to build, query, and manage MySQL databases effectively.
The course begins with An Introduction to MySQL, which covers the basics of relational databases, client/server systems, and the relational database model. You’ll explore SQL-based systems, including MySQL, and learn how to work with database objects, query data, and manipulate tables. This module sets the foundation for understanding MySQL’s architecture and how to interact with it through SQL.
In How to Use MySQL Workbench and Other Development Tools, you'll learn to navigate MySQL Workbench, the primary graphical user interface for MySQL. This module covers connecting to databases, running SQL statements, managing database objects, and using the MySQL Command Line Client. You’ll gain hands-on experience in executing and managing SQL scripts, making it easier to work with MySQL databases.
The How to Retrieve Data from a Single Table section introduces you to the SELECT statement, the cornerstone of SQL querying. You’ll learn to select specific columns, filter data with the WHERE clause, sort results, and use various SQL functions to manipulate data. This module helps you master querying techniques that form the core of everyday database operations.
In How to Retrieve Data from Two or More Tables, you'll explore advanced querying techniques, including inner joins, outer joins, and cross joins. You’ll learn to combine data from multiple tables using various join methods and work with unions to merge result sets. This module is essential for understanding how to query complex data relationships in MySQL.
The How to Insert, Update, and Delete Data module covers the data manipulation language (DML) aspects of SQL. You’ll learn to create test tables, insert new rows, update existing data, and delete records using subqueries and conditions. These skills are crucial for managing and maintaining data within your databases.
Next, in More SQL Skills as You Need Them, you'll delve into advanced SQL techniques, including summary queries, aggregate functions, and grouping data with the GROUP BY and HAVING clauses. This section also covers subqueries, common table expressions (CTEs), and working with complex queries, equipping you with the skills to handle sophisticated data analysis tasks.
In the Stored Program Development section, you’ll learn to develop stored procedures, functions, triggers, and events in MySQL. This module covers procedural coding skills, using transactions and locking for data consistency, and managing concurrency. You’ll also explore how to create and manage stored routines using MySQL Workbench, adding a powerful layer of functionality to your databases.
The course concludes with How to Use Functions, focusing on string, numeric, date/time, and other essential functions in MySQL. You’ll learn to format and manipulate data using built-in functions, enhancing your ability to perform complex calculations and data transformations directly within your SQL queries.
By the end of this course, you will have gained a thorough understanding of MySQL development, including how to query data, manage database structures, and develop stored programs. You’ll be equipped with the practical skills needed to build, maintain, and optimize MySQL databases, making you a valuable asset in any data-driven environment.
- Create views to reuse SELECT statements
- Learn data retrieval using SELECT statement.
- Troubleshoot typical warnings and errors.
- Change or add data.
- Understand MySQL data validation.
- Delete data from tables.
- Generate aggregated query data using various criteria.
- Connect data from multiple table rows using various types of JOIN constructs.
- Use several different types of sub-queries.
- Extensive coverage of MySQL Functions and expressions.
- Use expressions in SQL statements for more functional and flexible retrieval.
- Learn to export and import data.
- An introduction to MySQL
- An introduction to relational databases
- An introduction to client/server systems
- The hardware components of a client/server system
- The software components of a client/server system
- Other client/server architectures
- An introduction to the relational database model
- How a table is organized
- How tables are related
- How columns are defined
- How to read a database diagram
- An introduction to SQL and SQL-based systems
- A brief history of SQL
- A comparison of Oracle, DB2, Microsoft SQL Server, and MySQL
- The SQL statements
- An introduction to the SQL statements
- How to work with database objects
- How to query a single table
- How to join data from two or more tables
- How to add, update, and delete data in a table
- SQL coding guidelines
- How to use SQL from an application program
- Common options for accessing MySQL data
- PHP code that retrieves data from MySQL
- Java code that retrieves data from MySQL
- An introduction to client/server systems
- How to use MySQL Workbench and other development tools
- An introduction to MySQL Workbench
- The Home page of MySQL Workbench
- How to open a database connection
- How to start and stop the database server
- How to navigate through the database objects
- How to view and edit the data for a table
- How to view and edit the column definitions for a table
- How to use MySQL Workbench to run SQL statements
- How to enter and execute a SQL statement
- How to use snippets
- How to handle syntax errors
- How to open and save SQL scripts
- How to enter and execute SQL scripts
- How to use the MySQL Reference Manual
- How to view the manual
- How to look up information
- How to use the MySQL Command Line Client
- How to start and stop the MySQL Command Line Client
- How to use the MySQL Command Line Client to work with a database
- An introduction to MySQL Workbench
- How to retrieve data from a single table
- An introduction to the SELECT statement
- The basic syntax of the SELECT statement
- SELECT statement examples
- How to code the SELECT clause
- How to code column specifications
- How to name the columns in a result set using aliases
- How to code arithmetic expressions
- How to use the CONCAT function to join strings
- How to use functions with strings, dates, and numbers
- How to test expressions by coding statements without FROM clauses
- How to eliminate duplicate rows
- How to code the WHERE clause
- How to use the comparison operators
- How to use the AND, OR, and NOT logical operators
- How to use the IN operator
- How to use the BETWEEN operator
- How to use the LIKE and REGEXP operators
- How to use the IS NULL clause
- How to code the ORDER BY clause
- How to sort by a column name
- How to sort by an alias, expression, or column number
- How to code the LIMIT clause
- How to limit the number of rows
- How to return a range of rows
- An introduction to the SELECT statement
- How to retrieve data from two or more tables
- How to work with inner joins
- How to code an inner join
- How to use table aliases
- How to join to a table in another database
- How to use compound join conditions
- How to use a self-join
- How to join more than two tables
- How to use the implicit inner join syntax
- How to work with outer joins
- How to code an outer join
- Outer join examples
- Other skills for working with joins
- How to join tables with the USING keyword
- How to join tables with the NATURAL keyword
- How to use cross joins
- How to work with unions
- How to code a union
- A union that combines result sets from different tables
- A union that combines result sets from the same tables
- A union that simulates a full outer join
- How to work with inner joins
- How to insert, update, and delete data
- How to create test tables
- How to create the tables for this book
- How to create a copy of a table
- How to insert new rows
- How to insert a single row
- How to insert multiple rows
- How to insert default values and null values
- How to use a subquery in an INSERT statement
- How to update existing rows
- How to update rows
- How to use a subquery in an UPDATE statement
- How to delete existing rows
- How to delete rows
- How to use a subquery in a DELETE statement
- How to create test tables
- An introduction to relational databases
- More SQL skills as you need them
- How to code summary queries
- How to work with aggregate functions
- How to code aggregate functions
- Queries that use aggregate functions
- How to group and summarize data
- How to code the GROUP BY and HAVING clauses
- Queries that use the GROUP BY and HAVING clauses
- How the HAVING clause compares to the WHERE clause
- How to code compound search conditions
- How to use the WITH ROLLUP operator
- How to use the GROUPING function
- How to code aggregate window functions
- How the aggregate window functions work
- How to use frames
- How to use named windows
- How to work with aggregate functions
- How to code subqueries
- An introduction to subqueries
- Where to code subqueries
- When to use subqueries
- How to code subqueries in the WHERE clause
- How to use the IN operator
- How to use the comparison operators
- How to use the ALL keyword
- How to use the ANY and SOME keywords
- How to cde correlated subqueries
- How to use the EXISTS operator
- How to code subqueries in other clauses
- How to code subqueries in the HAVING clause
- How to code subqueries in the SELECT clause
- How to code subqueries in the FROM clause
- How to work with complex queries
- A complex query that uses subqueries
- A procedure for building complex queries
- How to work with common table expressions
- How to code a CTE
- How to code a recursive CTE
- An introduction to subqueries
- How to work with data types
- The data types
- Overview
- The character types
- The integer types
- The fixed-point and floating-point types
- The date and time types
- The ENUM and SET types
- The large object types
- How to convert data
- How implicit data conversion works
- How to convert data using the CAST and CONVERT functions
- How to convert data using the FORMAT and CHAR functions
- The data types
- How to use functions
- How to work with string data
- A summary of the string functions
- Examples that use string functions
- How to sort by a string column that contains numbers
- How to parse a string
- How to work with numeric data
- How to use the numeric functions
- How to search for floating-point numbers
- How to work with date/time data
- How to get the current date and time
- How to parse dates and times with date/time functions
- How to parse dates and times with the EXTRACT function
- How to format dates and times
- How to perform calculations on dates and times
- How to search for a date
- How to search for a time
- Other functions you should know about
- How to use the CASE function
- How to use the IF, IFNULL, and COALESCE functions
- How to use the regular expression functions
- How to use the ranking functions
- How to use the analytic functions
- How to work with string data
- How to code summary queries
- Stored program development
- Language skills for writing stored programs
- An introduction to stored programs
- Four types of stored programs
- A script that creates and calls a stored procedure
- A summary of statements for coding stored programs
- How to write procedural code
- How to display data
- How to declare and set variables
- How to code IF statements
- How to code CASE statements
- How to code loops
- How to use a cursor
- How to declare a condition handler
- How to use a condition handler
- How to use multiple condition handlers
- An introduction to stored programs
- How to use transactions and locking
- How to work with transactions
- How to commit and rollback transactions
- How to work with save points
- How to work with concurrency and locking
- How concurrency and locking are related
- The four concurrency problems that locks can prevent
- How to set the transaction isolation level
- How to lock selected rows
- How to prevent deadlocks
- How to work with transactions
- How to create stored procedures and functions
- How to code stored procedures
- How to create and call a stored procedure
- How to code input and output parameters
- How to set a default value for a parameter
- How to validate parameters and raise errors
- A stored procedure that inserts a row
- How to work with user variables
- How to work with dynamic SQL
- How to drop a stored procedure
- How to code stored functions
- How to create and call a function
- How to use function characteristics
- A function that calculates balance due
- How to drop a function
- How to use Workbench with procedures and functions
- How to view and edit stored routines
- How to create stored routines
- How to drop stored routines
- How to code stored procedures
- How to create triggers and events
- How to work with triggers
- How to create a BEFORE trigger
- How to use a trigger to enforce data consistency
- How to create an AFTER trigger
- How to view or drop triggers
- How to work with events
- How to turn the event scheduler on or off
- How to create an event
- How to view, alter, or drop events
- How to work with triggers
- Language skills for writing stored programs
Each student will receive a comprehensive set of materials, including course notes and all the class examples.
Experience in the following would be useful for this MySQL class:
- Some knowledge of database concepts.
Live Private Class
- Private Class for your Team
- Live training
- Online or On-location
- Customizable
- Expert Instructors