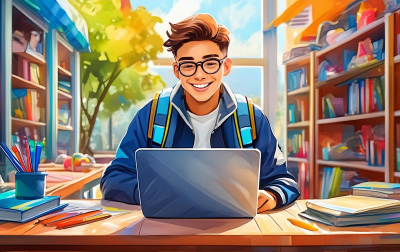
Programming with HTML, CSS, and JavaScript (HCJ501)
Course Length: Custom Length
Delivery Methods:
Available as private class only
Course Overview
Course Benefits
Class Materials
Each student will receive a comprehensive set of materials, including course notes and all the class examples.
Request a Private Class
- Private Class for your Team
- Online or On-location
- Customizable
- Expert Instructors