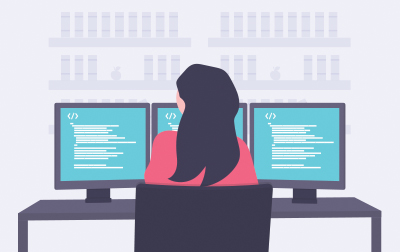
Object-Oriented Programming in C# (OBJ201)
This thorough and comprehensive Object-Oriented Programming in C# training class provides a practical introduction to programming in C#, utilizing the services provided by .NET. This course emphasizes the C# language.
This course is intended to be fully accessible to programmers who do not already have a strong background in object-oriented programming in C-like languages, such as C++ or Java. It is ideal, for example, for procedural programmers who desire to learn C#.
An important thrust of the course is to teach C# programming from an object-oriented perspective. It is often difficult for programmers trained originally in a procedural language to start "thinking in objects." This course introduces object-oriented concepts early, and C# is developed in a way that leverages its object orientation. A case study is used to illustrate creating a complete system using C# and .NET. Besides supporting traditional object-oriented features, such as classes, inheritance, and polymorphism, C# introduces several additional features, such as properties, indexers, delegates, events, and interfaces that make C# a compelling language for developing object-oriented and component-based systems. This course provides thorough coverage of all these features.
C# as a language is elegant and powerful. But to utilize its capabilities fully, you need to have a good understanding of how it works with the .NET Framework. The course explores several important interactions between C# and the .NET Framework, and it includes an introduction to major classes for collections, delegates, and events. It includes a succinct introduction to creating GUI programs using Windows Forms.
- Acquire a working knowledge of C# programming
- Learn how to implement programs using C# and classes from the .NET Framework
- Learn how to implement simple GUI programs using Windows Forms
- Gain a working knowledge of important newer features in C#
Public expert-led online training from the convenience of your home, office or anywhere with an internet connection. Guaranteed to run .
Private classes are delivered for groups at your offices or a location of your choice.
- Introduction to NET
- What is .NET?
- .NET Framework and .NET Core
- Application Models
- Managed Code
- Visual Studio 2019
- C# Console and GUI Programs
- First C# Programs
- Hello, World
- Namespaces
- Variables and Expressions
- Using C# as a Calculator
- Input/Output in C#
- .NET Framework Class Library
- Data Types in C#
- Data Types
- Integer Types
- Floating Point Types
- Decimal Type
- Characters and Strings
- Boolean Type
- Conversions
- Nullable Types
- Operators and Expressions
- Operator Cardinality
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Expressions
- Checked and Unchecked
- Control Structures
- If Tests
- Loops
- Arrays
- Foreach
- More about Control Flow
- Switch
- Object-Oriented Programming
- Objects
- Classes
- Inheritance
- Polymorphism
- Object-Oriented Languages
- Components
- Classes
- Classes as Structured Data
- Methods
- Constructors and Initialization
- Static Fields and Methods
- Constant and Readonly
- More about Types
- Overview of Types in C#
- Value Types
- Boxing and Unboxing
- Reference Types
- Implicitly Typed Variables
- Methods, Properties and Operators
- Methods
- Parameter Passing
- Method Overloading
- Variable-Length Parameter Lists
- Properties
- Auto-Implemented Properties
- Operator Overloading
- Characters and Strings
- Characters
- Strings
- String Input
- String Methods
- StringBuilder Class
- Programming with Strings
- Arrays and Indexers
- Arrays
- System.Array
- Random Number Generation
- Jagged Arrays
- Rectangular Arrays
- Arrays as Collections
- Bank Case Study—Step 1
- Indexers
- Inheritance
- Single Inheritance
- Access Control
- Method Hiding
- Initialization
- Bank Case Study—Step 2
- Virtual Methods and Polymorphism
- Virtual Methods and Dynamic Binding
- Method Overriding
- Fragile Base Class Problem
- Polymorphism
- Abstract Classes
- Sealed Classes
- Heterogeneous Collections
- Bank Case Study—Step 3
- Formatting and Conversion
- ToString
- Format Strings
- String Formatting Methods
- Bank Case Study—Step 4
- Type Conversions
- Exceptions
- Exception Fundamentals
- Structured Exception Handling
- User-Defined Exception Classes
- Inner Exceptions
- Bank Case Study—Step 5
- Interfaces
- Interface Fundamentals
- Programming with Interfaces
- Using Interfaces at Runtime
- Bank Case Study—Step 6
- Resolving Ambiguities
- .NET Interfaces and Collections
- Collections
- Bank Case Study—Step 7
- IEnumerable and IEnumerator
- Copy Semantics and ICloneable
- Comparing Objects
- Generic Types
- Type-Safe Collections
- Object Initializers
- Collection Initializers
- Anonymous Types
- Bank Case Study—Step 8
- Delegates and Events
- Delegates
- Anonymous Methods
- Lambda Expressions
- Events
- Introduction to Windows Forms
- Creating Windows Applications Using Visual Studio 2019
- Partial Classes
- Buttons, Labels and Textboxes
- Handling Events
- Listbox Controls
- Newer Features in C#
Each student will receive a comprehensive set of materials, including course notes and all the class examples.
Experience in the following is required for this C# class:
- Programming experience in a high-level language.
Live Public Class
$2,975.00 / student
Live Private Class
- Private Class for your Team
- Live training
- Online or On-location
- Customizable
- Expert Instructors